C++ 스터디 #14: 헤더파일
시간 | 2021년 8월 17일 화요일 20:00 ~ 22:00 |
장소 | ZOOM |
참가자 | - |
1. 헤더 파일
1.1 헤더 파일이란?
헤더 파일이란 컴파일러에 의해 다른 소스 파일에 자동으로 포함된 소스 코드의 파일을 말한다. 일반적으로 자주 사용하는 기능들을 기능들을 미리 함수로 선언하고 정의해 놓았다.
대표적으로 우리가 지금까지 써왔던 #include <iostream> 의 <iostream>도 cout, cin, endl 등의 기본 입출력과 관계된 객체들을 정리해 둔 헤더파일이다. <iostream> 은 컴파일러 제작사가 만들어 배포하는 '표준 라이브러리 디렉토리'에 존재한다.
1.2 std 접두어
표준 라이브러리에 있는 함수나 변수는 std 표준 네임스페이스에 포함되어 있다. 그래서 표준 라이브러리를 사용할때는 std:: 접두사를 붙여야 한다.
#include<iostream> int main() { std::cout << "Hello world!" << std::endl; return 0; }우리가 지금까지 사용했던
using namespace std;
는 std:: 접두사를 계속 붙이지 않기 위함이었다.
이를 이용하면 다음과 같이 코드를 바꿀 수 있다.
#include<iostream> using namespace std; int main() { cout << "Hello world!" << endl; return 0; }
2. 자주 사용하는 헤더 파일
2.1 iostream
지금까지 많이 써 왔던 iostream은 C++ 입출력 기본을 제공한다. Input/Output Stream(입출력 스트림)에서 이름을 따왔다. cin, cout, endl 등의 함수를 포함한다.
#include<iostream> using namespace std; int main() { cout << "Hello world!" << endl; return 0; }
2.2 string.h
string.h은 C++ 표준 문자열 클래스를 제공한다. 메모리 블록이나 문자열을 다룰 수 있는 함수들을 포함하고 있다.
#include<iostream> #include<string.h> using namespace std; int main() { char str1[] = "Hello, world!"; char str2[100]; strcpy_s(str2, str1); cout << str2 << endl; return 0; }
2.3 math.h
cmath는 기초 수식 함수들을 구현하는 C 프로그래밍 언어의 표준 라이브러리 안의 함수들의 모임이다.
#include<iostream> #include<math.h> using namespace std; int main() { int num1 = 5; int num2 = pow(num1, 2); cout << num2 << " " << sqrt(num2) << endl; return 0; }
2.4 time.h
time.h은 시간과 날짜를 얻거나 조작하는 함수들을 포함하고 있다.
#include <iostream> #include <stdlib.h> #include <time.h> using namespace std; int main() { cout << time(NULL) << endl; srand((unsigned int)time(NULL)); cout << rand() % 10 << endl; return 0; }
2.5 stdlib.h
stdlib.h는 동적 메모리 할당을 위한 수동 메모리 관리를 수행한다. malloc, free, new, delete 등의 함수를 포함한다.
#include<iostream> #include<stdlib.h> using namespace std; int main() { int* p; p = new int; delete p; return 0; }
2.6 windows.h
windows.h는 윈도우 개발자들이 필요한 모든 매크로들, 다양한 함수들과 서브시스템에서 사용되는 모든 데이터 타입들 그리고 윈도우 API의 함수들을 위한 정의를 포함하는 윈도우의 C 및 C++ 헤더 파일이다.
#include<iostream> #include<Windows.h> using namespace std; class Circle { public: Circle(int xval, int yval, int r); void draw(); void move(); private: int x, y, radius; }; Circle::Circle(int xval, int yval, int r) : x(xval), y(yval), radius(r) { } void Circle::draw() { HDC hdc = GetWindowDC(GetForegroundWindow()); Ellipse(hdc, x - radius, y - radius, x + radius, y + radius); } void Circle::move() { x += rand() % 50; } int main() { Circle c1(100, 100, 50); Circle c2(100, 200, 40); for (int i = 0; i < 20; i++) { c1.move(); c1.draw(); c2.move(); c2.draw(); Sleep(1000); } return 0; }
3. 원하는 헤더 파일 만들고 사용하기
3.1 개요
클래스를 외부에 정의하면 여러 소스 파일에서 이 클래스를 사용할 수 있다.
서로 다른 소스에서 같은 클래스를 사용하려 할때, 하나의 새롭게 클래스에 대한 모든 선언을 하는것은 힘들다. 따라서 클래스를 헤더 파일과 소스 파일로 나누어 작성하면 더 좋을 수 있다.
3.2 내가 만든 클래스를 헤더 파일로 사용하기
내가 만든 클래스를 헤더 파일로 사용하는 예를 Car 클래스를 통해 살펴보자.
<car.h>
#include<iostream> #include<string> using namespace std; class Car { int speed; int gear; string color; public : int getSpeed(); void setSpeed(int s); };먼저 이렇게 car.h 헤더파일을 선언해준다. car.h에는 클래스의 선언이 들어간다.
이후 멤버 함수 getSpeed()와 setSpeed(int s)는 별도의 소스 파일인 car.cpp에서 정의 할 수 있다. 이때, car.cpp에는 car.h를 반드시 포함하여야 하고 #include car.h 를 한 위치에 car.h가 들어가게 된다.
car.h는
#include “car.h”
로 포함할 수 있다. <car.cpp>
#include "car.h" int Car::getSpeed() { return speed; } void Car::setSpeed(int s) { speed = s; }
이후 다른 소스 파일에서 Car 클래스를 사용하려면 헤더 파일 car.h만 포함하면 된다. 새로운 소스 파일인 main.cpp에서 Car 클래스를 사용하는 것을 살펴보자.
<main.cpp>
#include "car.h" int main() { Car myCar; myCar.setSpeed(100); cout << "현재 속도는 " << myCar.getSpeed() << endl; return 0; }이들은 다음 사진과 같이 서로 다른 소스 파일이 된다.
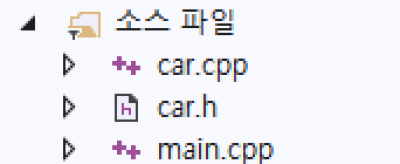
이렇게 클래스의 선언(car.h)과 구현(car.cpp)을 따로 함으로써 개발할 때 car.h를 여러 소스 파일에서 사용할 수 있게 되었다.